Unity On Collision
If any collision is detected along the ray, the object is moved to that position, the time is advanced to the collision time (which can be predicted using the collision distance), and then the process is iterated. This is a very simplified explanation, and you can find much more details on the internet.
In this tutorial, we will see how to detect collision in unity 3D between different game objects. We will use Collision class to detect the collisions between game objects.
We will use below scene for demo.
Unity is the ultimate game development platform. Use Unity to build high-quality 3D and 2D games, deploy them across mobile, desktop, VR/AR, consoles or the Web, and connect with loyal and enthusiastic players and customers. Since only fast-moving objects suffer from the tunnelling problem, developers often enable continuous collision detection only for these objects. In Unity, the option to turn on continuous collision detection can be found on the Rigidbody2D and Rigidbody components, which are used in 2D and 3D games respectively to give objects physics-based. Want a beginners guide to destroying an object upon collision in Unity3D? Well you found it.In just a couple of minutes I show you how you can destroy and du.

We will move player object with keyboard input and check it’s collision with other objects.
Steps to detect collision in Unity 3D
Step 1:
The game object on which collision detection will be triggered should be having Rigid Body and Collider components. For this demo, we will attach Rigid body component to the player game object. Box collider is already available to primitive Cube objects.
Make sure Is Kinematic check box is unchecked.
Step 2:
Attach Collider components to the other game objects. Here we have added Box Collider to Ground and Enemy Game Objects. Rigid Body component is optional. Make sure that “Is Trigger” checkbox is unchecked.
Step 3: Attach below script to Player game object.
2 4 6 8 10 12 14 16 18 20 22 24 | using UnityEngine.UI; publicclassCollisionBehavior:MonoBehaviour publicText LogCollsiionEnter; publicText LogCollisionExit; privatevoidOnCollisionEnter(Collision collision) LogCollsiionEnter.text='On Collision Enter: '+collision.collider.name; { LogCollisionStay.text='On Collision stay: '+collision.collider.name; { LogCollisionExit.text='On Collision exit: '+collision.collider.name; } |
Our setup is complete. Run the application to see the results.
Collide with specific objects – layers
We can also detect collision for specific set of objects. We can use layers for the same. For this example, we will do the following changes:
- Create three different layers, Ground, Player and Enemy. Apply these layers to respective game objects. To know more about layers, checkout this layer masking tutorial.
- Open Physics settings. (Edit -> Project settings -> Physics)
- There is Layer Collision Matrix in physics settings. Here we can check that which layer will interact with other layers.
With above settings Player Layer Objects with not collide with Enemy layered objects.
Hope you get an idea about to detect collision in unity 3D. Post your comments for queries and feedback. Thanks for reading.
The following two tabs change content below.- Using Transparent Material in Unity 3D - February 8, 2021
- Getting started with UI Toolkit : Unity 3D Tutorial - December 30, 2020
- Using Events in Unity 3D - May 2, 2020
Collision detection is required for all types of games. Detecting and utilizing the collision is implemented in a different manner in different game engines. Unity collider can be added to any game object as a component. Also, you need to understand how colliders and rigidbody work together to efficiently use Unity colliders. Without a basic understanding of how Unity Colliders work, it will be very difficult to code the game mechanics.
If you are totally new to Unity check out how to learn Unity.
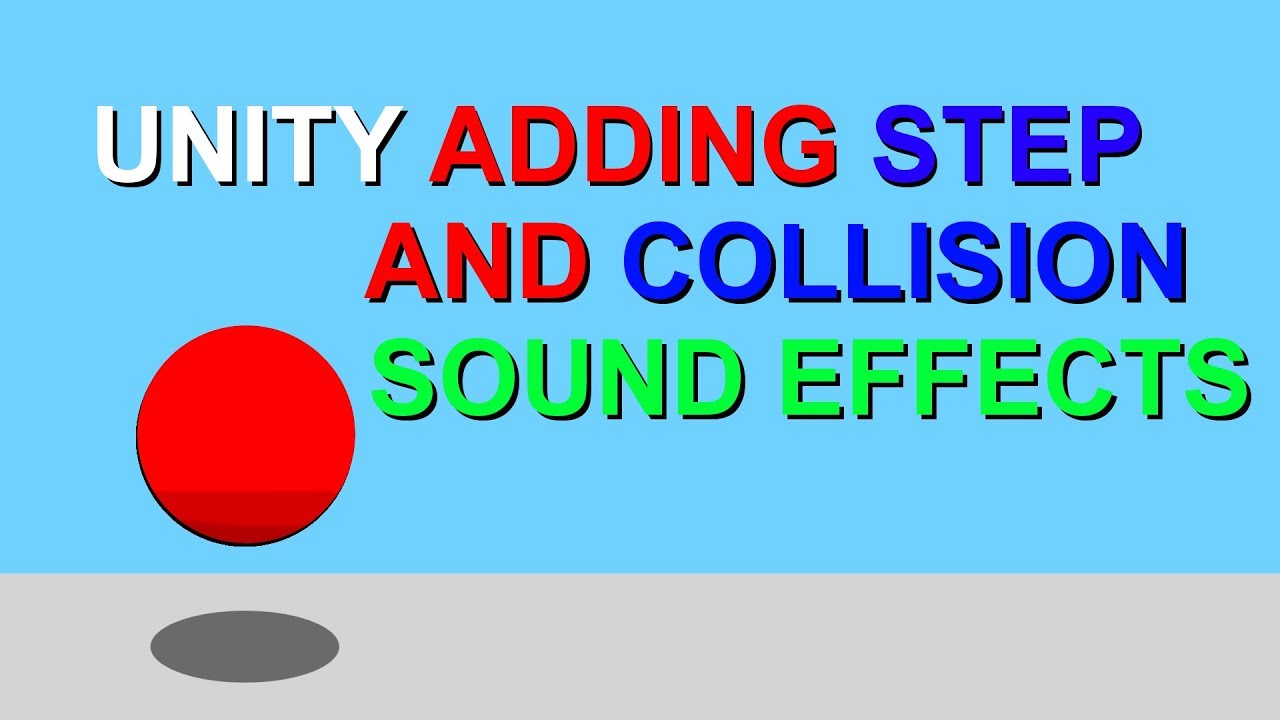
Unity colliders are very simple to use. Unity has divided the colliders into 2D and 3D. So you have to very careful which collider are you using. All your codes and collision detection will change for 2D and 3D. Further, there are types, static collider, rigidbody collider, kinematic rigidbody collider. The collider used in all these are the same, the type is for how the object to which the collider is added behaves.
Unity Collider Types
Destroy Object On Collision Unity
Static collider
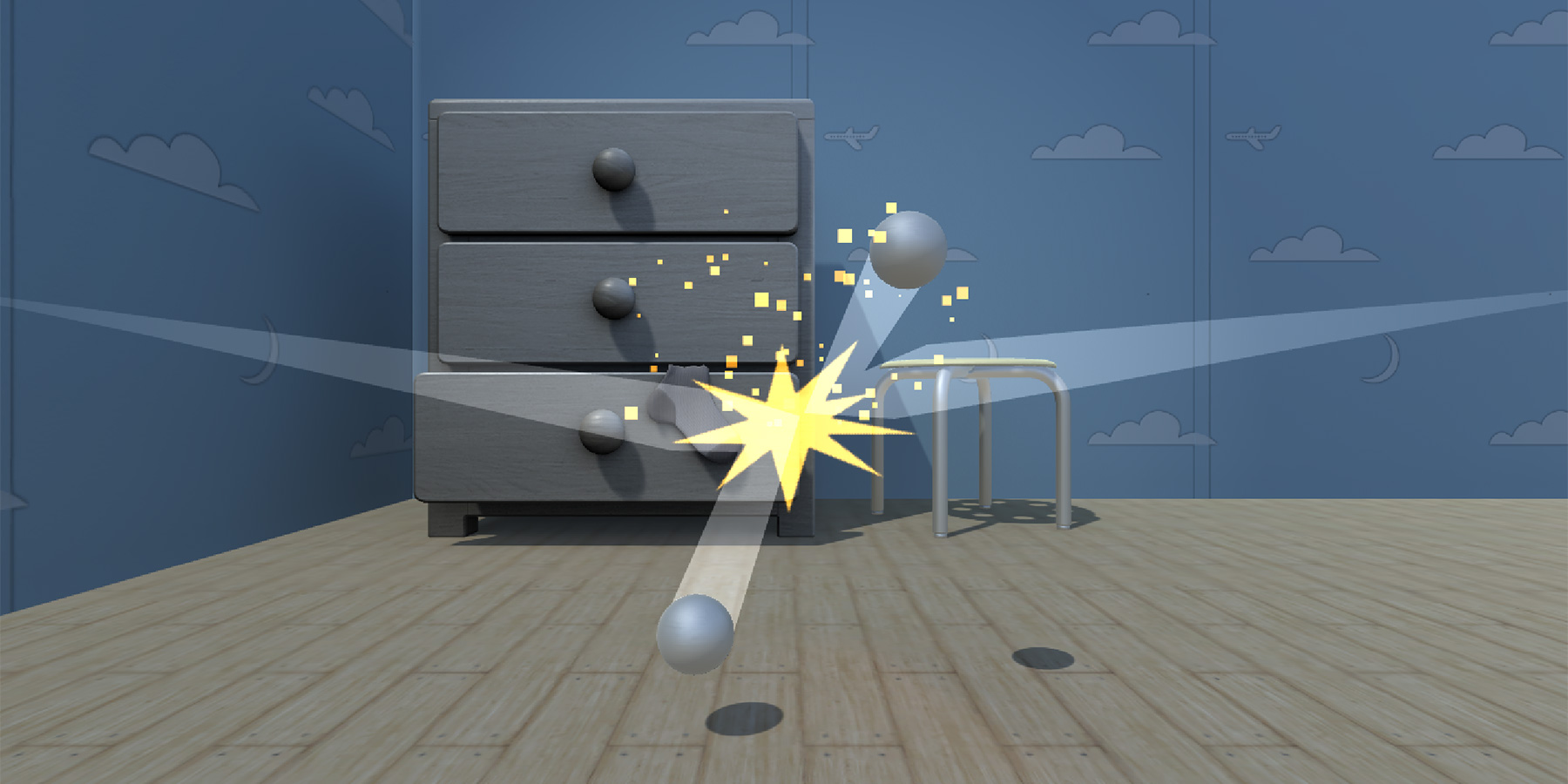
Static colliders are considered to be non-moving objects by unity. Do not confuse static gameobject with static collider. If a rigidbody is not attached to a collider then it’s a static collider. They do not move when an object Collides to with them. Though, they can be moved using transform, moving a static collider leads to performance loss during runtime.
Rigidbody collider
A rigidbody collider works like a real-world object. Apple free software for mac. It is governed by the forces of physics. If you apply force on it, it will move. In Unity, rigidbody is used on an object which will move a lot during gameplay. Check the screenshot for how a rigidbody is attached to a game object.
Kinematic rigidbody collider
A kinematic rigidbody is a rigidbody that behaves like a static object. So, the next question is then why to use a kinematic rigidbody. The main reason to use a kinematic rigidbody is to tell Unity that the object does not respond to forces but will be movable by script during runtime. The performance loss is very less in moving a kinematic rigidbody compared to a Static object.
OnCollisionEnter vs OnTriggerEnter
Unity onCollisionEnter
Unity will detect a collision only if one of the objects is a rigidbody. That is, if you have two gameobject marked as static colliders then collision between them will not be detected. It’s the same case with two kinematic rigidbody. If you use the OnCollisionEnter function in any of the above cases, the function will not be called during a Collision. Find the collision matrix for unity colliders in the image below.
Ref:unity docs
Unity OnTriggerEnter

When you mark a collider as a trigger, it no longer behaves like a solid object. It allows the other object to pass through it. But the time of one object entering another object’s space can trigger a function. With that, you can know if a collision has happened without actually creating a collision. You have to use the OnTriggerEnter function if the collider is Marked as a trigger. The collision matrix is a little different in the case of triggers.
Trigger collision doesn’t work if both the colliders are static colliders. In all the other cases the on trigger enter is called.Knowing these basic things about colliders will help you set them up easily in your game.
If you did not understand the matrix completely then here is a simple comparison to help you. Consider static colliders as object that don’t move like wall. Rigidbody collider denotes a moving object like a ball. Kinematic Rigidbody denotes that the object is not moved by physics but can be moved by script or animation.
Unity does not want to detect collision between two static objects so normal collision is detected only if one of the game object is a movable by physics. Where as trigger function works a little different. It works only if one of the collider is marked as trigger and can be moved either by physics or script.
Sample Unity collision script
unity OnCollisionEnter
In this script we are using a normal collision. “col” returns the collider of the game object. We further check if the game object is an enemy. If yes, we destroy it. Remember either the parent gameobject or the enemy game object needs to have a Rigidbody for this script to work.
Unity OnTriggerEnter
Unity On Collision Destroy
We can do the same thing with trigger with this code.
Pro tip: If the object is marked as trigger, it will pass through the obstacle and no collision physics will act.
Other features in Unity OnCollisionEnter and OntriggerEnter
Both the examples of Unity OnCollisionEnter and OnTriggerEnter above shows that you can get the gameobject of the colliding object. Apart from that you can get a lot of data from the collision class object. Here is the list of things that you can get from the collision class
- collider- get the collider of the colliding object
- relative velocity- get the relative velocity between the colliding objects.
- transform- get the transform of the object hit.
